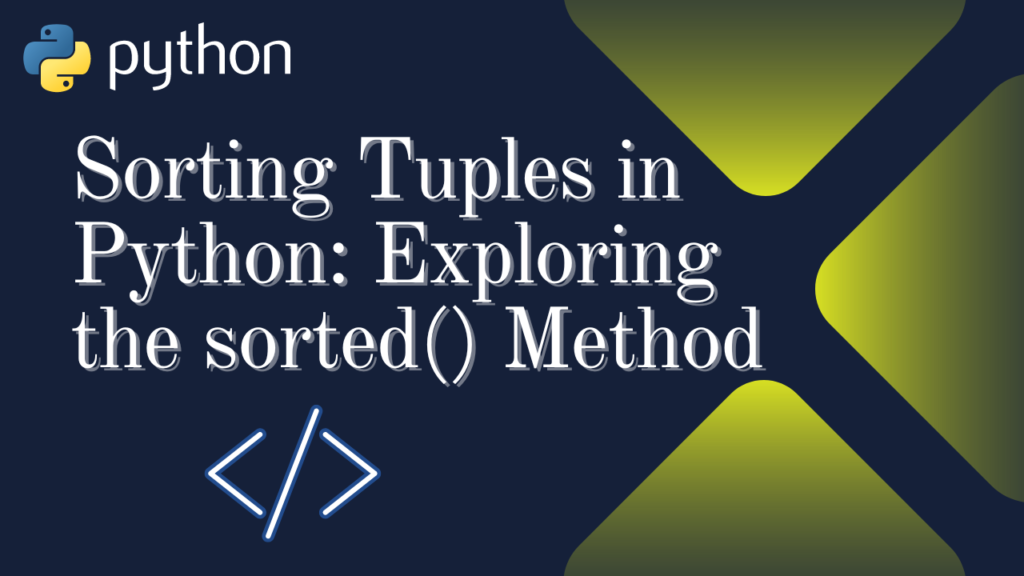
Introduction:
Python’s tuple data structure offers a variety of methods for efficient tuple manipulation. Among these methods, sorted() stands out as a powerful tool for sorting the elements within a tuple. In this blog post, we will explore the sorted() method in detail, uncovering its functionality, use cases, and providing practical examples to help you understand how to leverage it effectively for tuple sorting.
Understanding sorted() for Tuples:
The sorted() method in Python is used to sort the elements within a tuple in a specific order. It returns a new list containing the sorted elements.
Syntax:
The syntax for using the sorted() method is as follows:
sorted_list = sorted(tuple_name, key=None, reverse=False)
Here, tuple_name
represents the name of the tuple that we want to sort, key
is an optional parameter that specifies a function to customize the sort order, and reverse
is another optional parameter that indicates whether to sort the tuple in descending order.
Sorting a Tuple:
The sorted() method provides a convenient way to sort the elements within a tuple. Let’s explore some examples to illustrate its usage:
Example 1: Sorting a Tuple in Ascending Order
numbers = (5, 2, 8, 1, 7)
sorted_numbers = sorted(numbers)
print(sorted_numbers)
Output:
[1, 2, 5, 7, 8]
In this example, we have a tuple of numbers, and by calling the sorted() method, we obtain a new list sorted_numbers
that contains the sorted elements in ascending order.
Example 2: Sorting a Tuple in Descending Order
fruits = ("apple", "banana", "orange")
sorted_fruits = sorted(fruits, reverse=True)
print(sorted_fruits)
Output:
["orange", "banana", "apple"]
Here, we have a tuple of fruits, and by using the sorted() method with the reverse=True
argument, we obtain a new list sorted_fruits
with the elements sorted in descending order.
Example 3: Sorting a Tuple with a Custom Key Function
def get_length(word):
return len(word)
words = ("cat", "dog", "elephant", "bird")
sorted_words = sorted(words, key=get_length)
print(sorted_words) # Output: ["cat", "dog", "bird", "elephant"]
Output:
["cat", "dog", "bird", "elephant"]
In this example, we have a tuple of words, and we define a custom key function get_length()
that returns the length of each word. By using the sorted() method with the key
parameter set to the get_length
function, we obtain a new list sorted_words
with the words sorted based on their lengths.
Important Considerations:
- The sorted() method creates a new list containing the sorted elements and does not modify the original tuple.
- The sorted() method works for tuples of any type, including numeric tuples, string tuples, and tuples containing custom objects.
- When sorting custom objects, you can provide a
key
function that specifies the attribute or value to consider during the sorting process.
Tips for Effective Usage:
- Use the sorted() method to arrange the elements within a tuple in a desired order, allowing for easier searching, analysis, or presentation of the data.
- If you want to preserve the original tuple while creating a sorted version, assign the result of the sorted() method to a new variable.
Conclusion:
The sorted() method in Python is a powerful tool for sorting the elements within a tuple. By understanding its syntax, usage, and important considerations, you now possess a valuable tool for effective tuple sorting. Utilize the sorted() method effectively, and you’ll have greater control over arranging and organizing your tuples in Python.
Happy coding!
The Education Machine
Leave a Reply