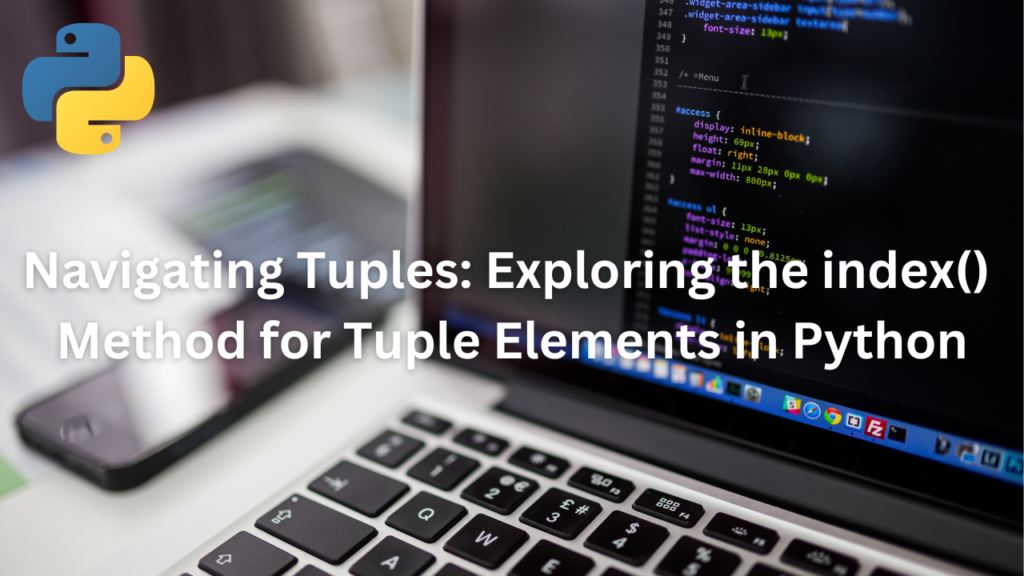
Introduction:
Python’s tuple data structure offers a variety of methods for efficient tuple manipulation. One such method is index(), which allows us to find the index of a specific element within a tuple. In this blog post, we will delve into the index() method for tuples, exploring its functionality, use cases, and providing practical examples to help you harness its power for effective tuple navigation.
Understanding index() for Tuples:
The index() method in Python is used to find the index of a specified element within a tuple. It returns the index of the first occurrence of the element.
Syntax:
The syntax for using the index() method is as follows:
tuple_name.index(element)
Here, tuple_name
represents the name of the tuple in which we want to find the element, and element
denotes the value we wish to locate.
Finding the Index of an Element within a Tuple:
The index() method enables us to retrieve the index of a specific element within a tuple, providing valuable information about its position. Let’s explore some examples to illustrate its usage:
Example 1: Finding the Index of an Element
fruits = ("apple", "banana", "orange")
index = fruits.index("banana")
print(index)
Output:
1
In this example, we have a tuple of fruits, and by calling the index() method with the element “banana”, we retrieve its index, which is 1. The index represents the first occurrence of the element within the tuple.
Example 2: Handling Value Error
colors = ("red", "blue", "green")
try:
index = colors.index("yellow")
print(index)
except ValueError:
print("Element not found.")
Here, we attempt to find the index of the element “yellow” within the tuple of colors. Since “yellow” is not present in the tuple, the index() method raises a ValueError. We handle the exception gracefully by printing a user-friendly error message.
Important Considerations:
- The index() method for tuples returns the index of the first occurrence of the specified element within the tuple.
- If the element is not found, the index() method raises a ValueError. Make sure to handle this exception appropriately.
- The index() method does not allow for specifying optional parameters like start or end indices, as tuples are immutable and do not support slicing.
Tips for Effective Usage:
- Before using the index() method, ensure that the element exists in the tuple by either checking its presence with the “in” keyword or handling the ValueError exception.
- Utilize the index() method to quickly locate the position of an element within a tuple, allowing for efficient tuple navigation.
Conclusion:
The index() method in Python provides a powerful tool for finding the index of a specific element within a tuple. By understanding its syntax, usage, and important considerations, you now possess a valuable tool for effective tuple navigation. Utilize the index() method effectively, and you’ll have greater control over accessing and manipulating elements within your tuples in Python.
Happy coding!
The Education Machine
Leave a Reply