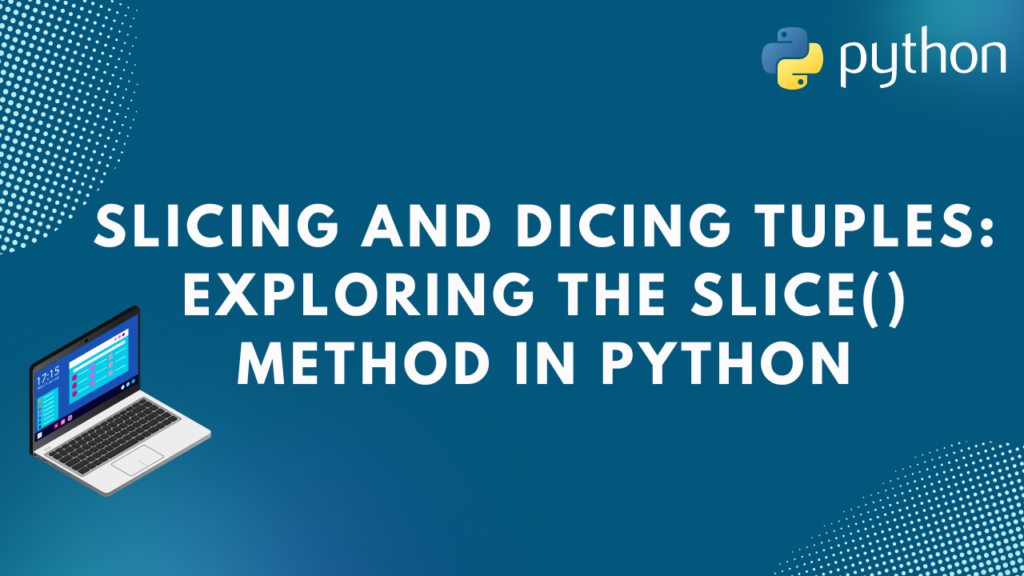
Introduction:
Python’s tuple data structure offers a range of methods for efficient tuple manipulation. Among these methods, slice() stands out as a powerful tool for extracting a subset of elements from a tuple. In this blog post, we will delve into the slice() method in detail, uncovering its functionality, use cases, and providing practical examples to help you understand how to leverage it effectively for tuple slicing.
Understanding slice() for Tuples:
The slice() method in Python is used to create a slice object that defines a range of indices for extracting a subset of elements from a tuple. It provides a flexible way to access specific portions of a tuple without modifying the original tuple.
Syntax:
The syntax for using the slice() method is as follows:
slice_object = slice(start, stop, step)
Here, start
represents the starting index of the slice (inclusive), stop
represents the ending index (exclusive), and step
represents the step size for selecting elements within the slice. All three parameters are optional.
Slicing Tuples:
The slice() method provides a convenient way to extract a subset of elements from a tuple based on specified indices. Let’s explore some examples to illustrate its usage:
Example 1: Basic Tuple Slicing
numbers = (1, 2, 3, 4, 5)
slice_obj = slice(1, 4)
sliced_tuple = numbers[slice_obj]
print(sliced_tuple)
Output:
(2, 3, 4)
In this example, we have a tuple of numbers, and by using the slice() method, we create a slice object slice_obj
that defines the range of indices from 1 to 4 (exclusive). We then use this slice object to extract the corresponding subset of elements from the original tuple, resulting in a new tuple sliced_tuple
with values (2, 3, 4).
Example 2: Slicing with Step Size
numbers = (1, 2, 3, 4, 5)
slice_obj = slice(0, 5, 2)
sliced_tuple = numbers[slice_obj]
print(sliced_tuple)
Output:
(1, 3, 5)
Here, we again have a tuple of numbers, and we use the slice() method to define a range of indices from 0 to 5 (exclusive) with a step size of 2. By applying this slice object to the original tuple, we obtain a new tuple sliced_tuple
containing the elements (1, 3, 5).
Example 3: Slicing with Negative Indices
fruits = ("apple", "banana", "orange", "grape", "kiwi")
slice_obj = slice(-3, None)
sliced_tuple = fruits[slice_obj]
print(sliced_tuple)
Output:
("orange", "grape", "kiwi")
In this example, we have a tuple of fruits, and we use the slice() method with a negative starting index (-3) and no ending index. This slice object defines a range from the third-last element to the end of the tuple. By applying this slice object to the original tuple, we obtain a new tuple sliced_tuple
with the values (“orange”, “grape”, “kiwi”).
Important Considerations:
- The slice() method creates a slice object that represents a range of indices within a tuple.
- The indices specified in the slice object are inclusive for the starting index and exclusive for the ending index.
- The step size parameter determines the number of elements to skip during slicing.
Tips for Effective Usage:
- Utilize the slice() method to extract specific portions of a tuple based on desired indices.
- Combine the slice object with tuple indexing or slicing operations to extract the subset of elements.
Conclusion:
The slice() method in Python is a powerful tool for slicing and extracting a subset of elements from a tuple based on specified indices. By understanding its syntax, usage, and important considerations, you now possess a valuable tool for effective tuple slicing. Utilize the slice() method effectively, and you’ll have greater control over accessing and manipulating specific portions of your tuples in Python.
Happy coding!
The Education Machine
Leave a Reply