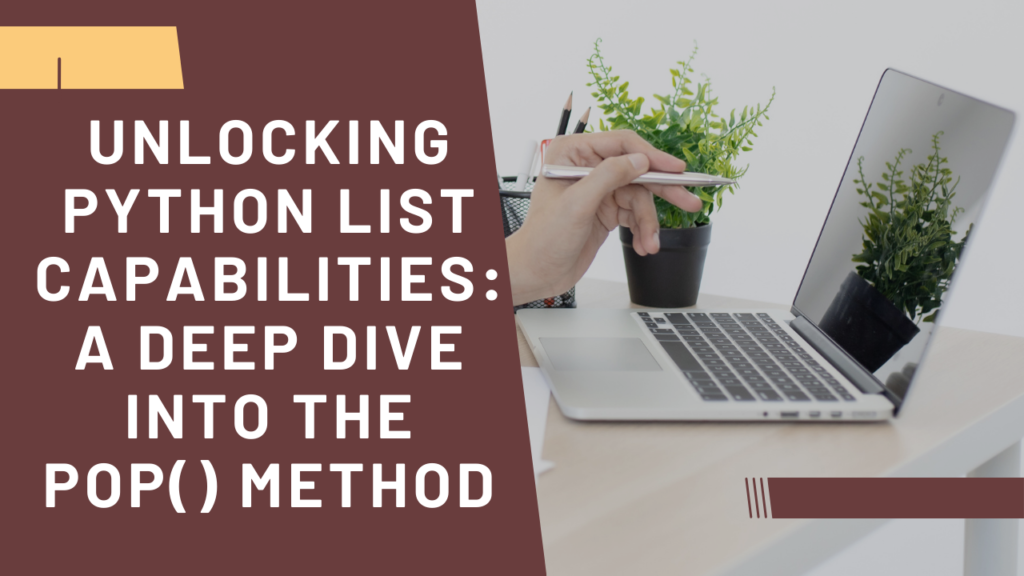
Introduction:
Python lists are versatile data structures that offer various methods for efficient list manipulation. One such method is pop(), which allows us to remove and retrieve elements from a list. In this blog post, we will explore the pop() method in detail, uncovering its functionality, use cases, and providing practical examples to help you harness its power for effective list operations.
Understanding pop():
The pop() method in Python removes and returns an element from a list at a specified index position. It allows us to modify the list by eliminating specific elements and retrieve their values simultaneously.
Syntax:
The syntax for using the pop() method is as follows:
list_name.pop(index)
Here, list_name
represents the name of the list from which we want to remove the element, and index
denotes the position of the element to be removed. The index is an optional parameter, with the default value being -1 (the last element).
Removing and Retrieving Elements:
The pop() method serves as a versatile tool for removing and retrieving elements from a list, providing flexibility and control over list operations. Let’s explore a few examples to illustrate its usage:
Example 1: Removing and Retrieving the Last Element
fruits = ["apple", "banana", "orange"]
last_fruit = fruits.pop()
print(fruits)
print(last_fruit)
Output:
["apple", "banana"]
"orange"
In this example, we have a list of fruits, and by calling the pop() method without specifying an index, we remove and retrieve the last element, “orange”. The updated list, fruits
, no longer contains the popped element.
Example 2: Removing and Retrieving an Element at a Specific Index
numbers = [1, 2, 3, 4]
second_number = numbers.pop(1)
print(numbers)
print(second_number)
Output:
[1, 3, 4]
2
Here, we have a list of numbers, and by specifying an index of 1 in the pop() method, we remove and retrieve the element at that position, which is 2. The resulting list, numbers
, is modified accordingly.
Example 3: Removing and Handling Index Error
colors = ["red", "blue", "green"]
try:
color = colors.pop(3)
print(color)
except IndexError:
print("Index out of range.")
In this case, we attempt to remove an element at index 3, which exceeds the valid range of the list. Since an IndexError occurs, we handle it gracefully by printing a user-friendly error message. It is crucial to handle such exceptions to prevent program crashes.
Important Considerations:
- The pop() method modifies the list in place. It does not create a new list but instead updates the existing one. Therefore, it’s essential to use pop() when we want to modify the original list.
- If the index is not specified, the pop() method removes and returns the last element by default. It effectively reduces the list’s size by one.
- When specifying an index, it should be within the valid range of the list. Otherwise, an IndexError will occur.
Tips for Effective Usage:
- To remove an element from a list without retrieving its value, you can simply use the del statement followed by the list index. This approach is more efficient than using pop() when the returned value is not required.
- To retrieve and remove multiple elements from a list, you can use pop() in a loop. Iterate through the desired indexes and pop() the elements one by one.
Conclusion:
The pop() method in Python provides a powerful mechanism for removing and retrieving elements from a list, allowing for efficient list manipulation. By understanding its syntax, usage, and important considerations, you now possess a valuable tool for precise list operations. Utilize the pop() method effectively, and you’ll have greater control over the composition and structure of your lists in Python.
Happy coding!
The Education Machine
Leave a Reply