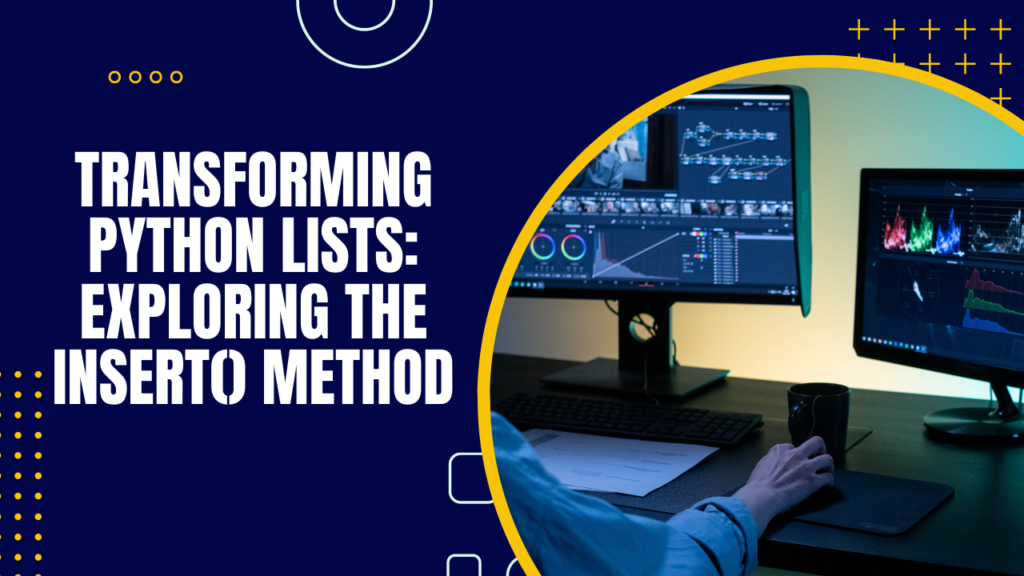
Introduction:
Python’s list data structure provides a plethora of powerful methods for manipulating and modifying lists. One such method is insert(), which allows us to insert elements at specific positions within a list. In this blog post, we will explore the insert() method in detail, unraveling its functionality, use cases, and practical examples to help you leverage its potential for precise list manipulation.
Understanding insert():
The insert() method in Python enables us to add an element at a specified index position within a list. It shifts the existing elements to accommodate the new element, expanding the list’s size as necessary.
Syntax:
The syntax for using the insert() method is as follows:
list_name.insert(index, element)
Here, list_name
represents the name of the list in which we want to insert the element, index
denotes the desired position, and element
is the value we wish to insert.
Inserting Elements at Specific Positions:
The insert() method allows us to place elements precisely within a list by specifying the desired index position. Let’s consider a few examples to illustrate its usage:
Example 1: Inserting at the Beginning of a List
fruits = ["apple", "banana", "orange"]
fruits.insert(0, "grape")
print(fruits)
Output:
["grape", "apple", "banana", "orange"]
In this example, we start with a list of fruits and use the insert() method to add the element “grape” at index 0. As a result, “grape” is inserted at the beginning of the list, pushing the existing elements one position to the right.
Example 2: Inserting in the Middle of a List
numbers = [1, 2, 3, 4]
numbers.insert(2, 10)
print(numbers)
Output:
[1, 2, 10, 3, 4]
Here, we have a list of numbers, and we use the insert() method to insert the value 10 at index 2. As a result, the element 10 is placed between the existing elements 2 and 3
Example 3: Inserting at the End of a List
colors = ["red", "blue", "green"]
colors.insert(len(colors), "yellow")
print(colors)
Output:
["red", "blue", "green", "yellow"]
In this case, we have a list of colors, and we use the insert() method to add the element “yellow” at the end of the list. By passing the length of the list as the index, we ensure that the element is inserted at the last position.
Important Considerations:
- The insert() method modifies the list in place. It does not create a new list but instead updates the existing one. Therefore, it’s essential to use insert() when we want to modify the original list.
- The index specified in the insert() method should be within the valid range of the list. Otherwise, it will result in an IndexError.
- The time complexity of the insert() method is O(n), where n is the number of elements in the list. Inserting an element at the beginning or middle of the list requires shifting subsequent elements, resulting in a potentially costly operation for large lists.
Tips for Effective Usage:
- Consider using append() instead of insert() when you need to add elements to the end of a list. The append() method is optimized for appending elements and generally performs better in such scenarios.
- When inserting elements at the beginning or middle of large lists frequently, consider using other data structures like deque, which provide efficient insertion and deletion at both ends.
Conclusion:
The insert() method in Python empowers us to add elements at specific positions within a list, expanding our list manipulation capabilities. By understanding its syntax, usage, and important considerations, you now have a powerful tool at your disposal for precise list modifications. Use the insert() method effectively, and you’ll have greater control over the structure and content of your lists in Python.
Happy coding!
The Education Machine
Leave a Reply