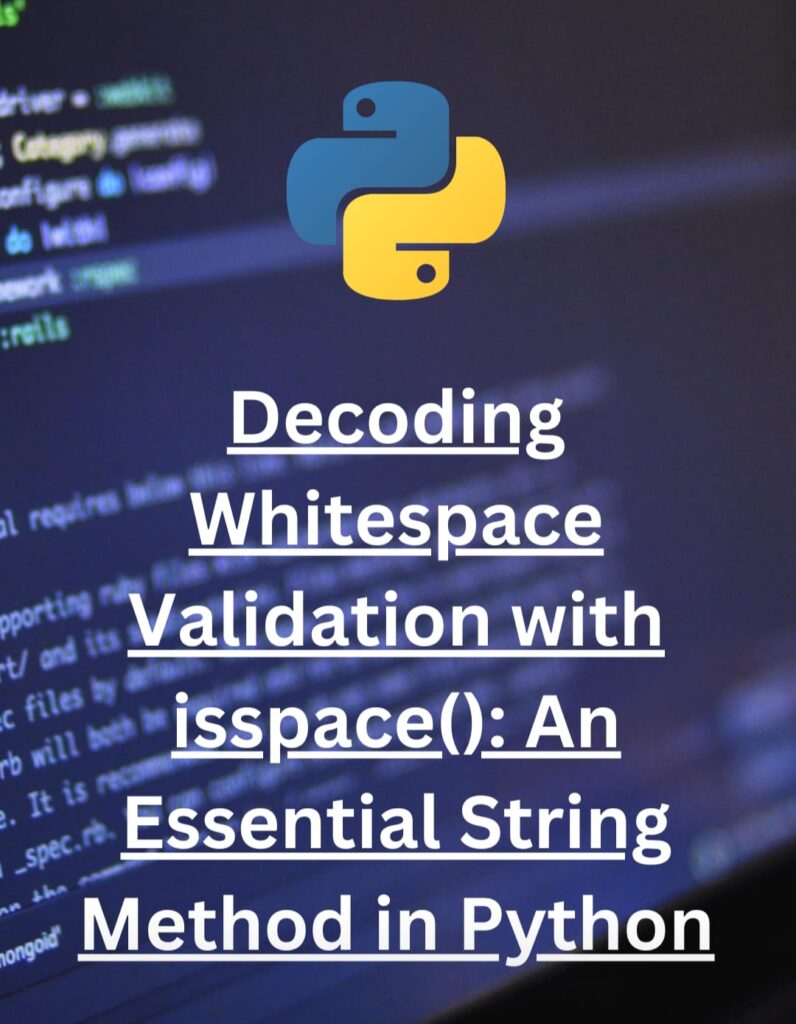
Introduction:
String manipulation is a fundamental aspect of Python programming, and Python provides a diverse set of built-in methods to handle strings effectively. Among these methods, isspace()
plays a crucial role in validating whether a string consists solely of whitespace characters. In this blog post, we will explore the isspace()
string method in Python, understand its functionality, and provide illustrative examples to demonstrate its usage.
The isspace()
method in Python is used to determine if a string contains only whitespace characters. It returns True
if all characters in the string are whitespace (spaces, tabs, newlines, etc.) and False
otherwise. The method ignores non-whitespace characters.
Syntax:
The syntax for the isspace()
method is as follows:
string.isspace()
Now, let’s delve into practical examples to understand how the isspace()
method works.
Example 1:
Basic Usage
text = " "
result = text.isspace()
print(result)
Output:
True
Explanation: In this example, the isspace()
method is used to validate the string " "
. Since all characters in the string are whitespace, the method returns True
.
Example 2:
Including Non-Whitespace Characters
text = " Hello "
result = text.isspace()
print(result)
Output:
False
Explanation: In this example, the isspace()
method is used to check the string " Hello "
. Since the string contains non-whitespace characters, such as the letter “H,” the method returns False
. It only considers whitespace characters and ignores any non-whitespace characters.
Example 3:
Empty String
text = ""
result = text.isspace()
print(result)
Output:
False
Explanation: In this example, the isspace()
method is used to validate an empty string. As an empty string does not contain any characters, including whitespace, the method returns False
.
Example 4:
Mixed Whitespace and Non-Whitespace Characters
text = "\t \nHello \t\n"
result = text.isspace()
print(result)
Output:
False
Explanation: In this example, the isspace()
method is used to validate the string "\t \nHello \t\n"
. Although the string contains whitespace characters (tabs and newlines), it also includes non-whitespace characters (the letter “H”). Thus, the method returns False
.
Conclusion:
The isspace()
method in Python is a valuable tool for validating whether a string consists solely of whitespace characters. By utilizing this method, you can easily ensure that a string meets specific whitespace-related constraints. Understanding the behavior and usage of the isspace()
method allows you to develop robust string validation mechanisms in Python. Incorporate the isspace()
method into your Python programs to enhance the reliability and integrity of string manipulation tasks, particularly when dealing with input validation or formatting. Experiment with different strings, including mixed whitespace and non-whitespace characters, to fully explore the capabilities of the isspace()
method and enhance your Python programming skills.
Happy Coding!
The Education Machine
Leave a Reply