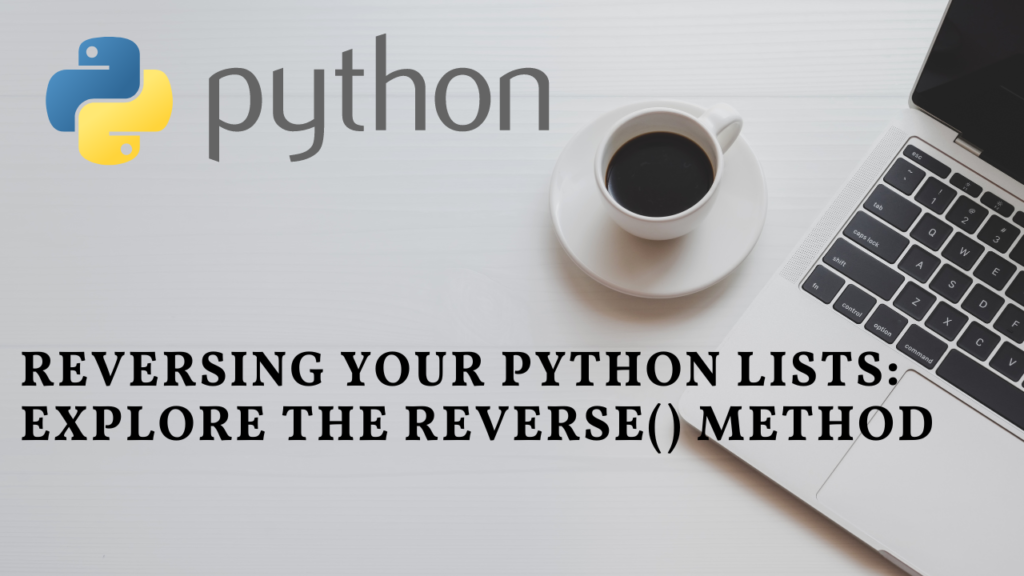
Introduction:
Python’s list data structure provides a range of methods for efficient list manipulation. Among these methods, reverse() stands out as a useful tool for reversing the order of elements within a list. In this blog post, we will explore the reverse() method in detail, uncovering its functionality, use cases, and providing practical examples to help you harness its power for effective list reversal.
Understanding reverse():
The reverse() method in Python allows us to reverse the order of elements within a list. It modifies the list in place, without creating a new list.
Syntax:
The syntax for using the reverse() method is as follows:
list_name.reverse()
Here, list_name
represents the name of the list that we want to reverse.
Reversing the Order of Elements:
The reverse() method provides a convenient way to reverse the order of elements within a list. Let’s explore some examples to illustrate its usage:
Example 1: Reversing the Order of a List
numbers = [1, 2, 3, 4, 5]
numbers.reverse()
print(numbers)
Output:
[5, 4, 3, 2, 1]
In this example, we have a list of numbers, and by calling the reverse() method, we reverse the order of the elements within the list.
Example 2: Reversing the Order of a String List
fruits = ["apple", "banana", "orange"]
fruits.reverse()
print(fruits)
Output:
["orange", "banana", "apple"]
Here, we have a list of fruits represented as strings, and by using the reverse() method, we reverse the order of the elements in the list.
Example 3: Reversing the Order of a Custom Object List
class Person:
def __init__(self, name):
self.name = name
people = [Person("Alice"), Person("Bob"), Person("Charlie")]
people.reverse()
for person in people:
print(person.name)
Output:
Charlie
Bob
Alice
In this example, we have a list of custom Person
objects, and by calling the reverse() method, we reverse the order of the objects within the list.
Important Considerations:
- The reverse() method modifies the list in place. It does not create a new reversed list but updates the existing list.
- The reverse() method works for lists of any type, including numeric lists, string lists, and lists containing custom objects.
- When reversing a list, the order of the elements is completely reversed, with the first element becoming the last and vice versa.
Tips for Effective Usage:
- Use the reverse() method to easily change the order of elements within a list, providing a different perspective on the data.
- If you want to create a reversed version of the list without modifying the original list, you can use the slicing technique with a step value of -1 (
list_name[::-1]
).
Conclusion:
The reverse() method in Python is a powerful tool for reversing the order of elements within a list. By understanding its syntax, usage, and important considerations, you now possess a valuable tool for effective list reversal. Utilize the reverse() method effectively, and you’ll have greater control over the arrangement and organization of your lists in Python.
Happy coding!
The Education Machine
Leave a Reply