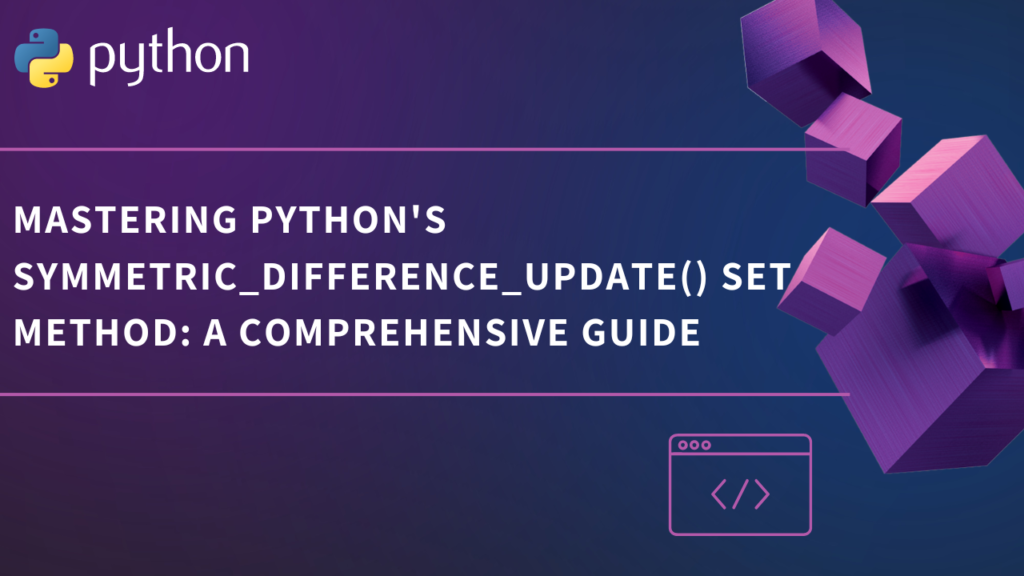
Introduction
In Python, the symmetric_difference_update() method is a powerful tool available for sets, allowing us to update a set with the elements that exist in either of two sets, but not in both. By leveraging this method, we can efficiently perform set operations, modify sets in place, and analyze differences between sets. In this comprehensive guide, we will delve into the intricacies of the symmetric_difference_update() method, exploring its functionality, syntax, usage examples, and best practices.
Understanding the symmetric_difference_update() Method
The symmetric_difference_update() method is designed specifically for sets in Python. It modifies the set on which the method is called by updating it with the elements that exist in either of two sets, but not in both. This method allows us to efficiently update sets in place, eliminating redundant elements and analyzing differences between sets.
Syntax of symmetric_difference_update()
The syntax for using the symmetric_difference_update() method is as follows:
set1.symmetric_difference_update(set2)
Here, set1 is the set on which the method is called, and set2 is the set to be compared. The method updates set1 with the symmetric difference between the two sets.
Exploring Examples
Let’s consider a few examples to better understand the usage and behavior of the symmetric_difference_update() method.
Example 1
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
set1.symmetric_difference_update(set2)
print(set1)
Output
{1, 2, 5, 6}
In this example, set1 and set2 have common elements 3 and 4. The symmetric_difference_update() method updates set1 by replacing its elements with the elements that are present in either set1 or set2, but not in both.
Example 2
set1 = {1, 2, 3}
set2 = {4, 5, 6}
set1.symmetric_difference_update(set2)
print(set1)
Output
{1, 2, 3, 4, 5, 6}
Here, set1 and set2 have no common elements. Therefore, the symmetric_difference_update() method updates set1 by adding all the elements from set2 that are not present in set1.
Common Use Cases
The symmetric_difference_update() method finds application in various scenarios, such as:
- Updating a set to contain the elements that exist in either of two sets, but not in both
- Removing redundant elements from a set and focusing on unique values
- Analyzing differences between sets or collections and updating them accordingly
- Efficiently modifying sets in place without creating new set objects
Best Practices for Using symmetric_difference_update()
To ensure optimal usage of the symmetric_difference_update() method, consider the following best practices:
- Utilize sets when appropriate, as they provide faster membership tests and eliminate duplicates.
- Combine the symmetric_difference_update() method with other set methods like union_update() and intersection_update() for advanced set operations.
- Make sure to store a copy of the original set if preservation of the original data is required.
Conclusion
In this comprehensive guide, we have explored the symmetric_difference_update() method in Python sets, understanding its purpose, syntax, and examples. We have also examined common use cases and provided best practices for efficient utilization. By leveraging the power of symmetric_difference_update(), you can perform set operations, modify sets in place, and analyze differences between sets in your Python programs.
Remember to experiment with the symmetric_difference_update() method and incorporate it into your Python projects to take full advantage of its capabilities.
Happy coding!
The Education Machine
Leave a Reply