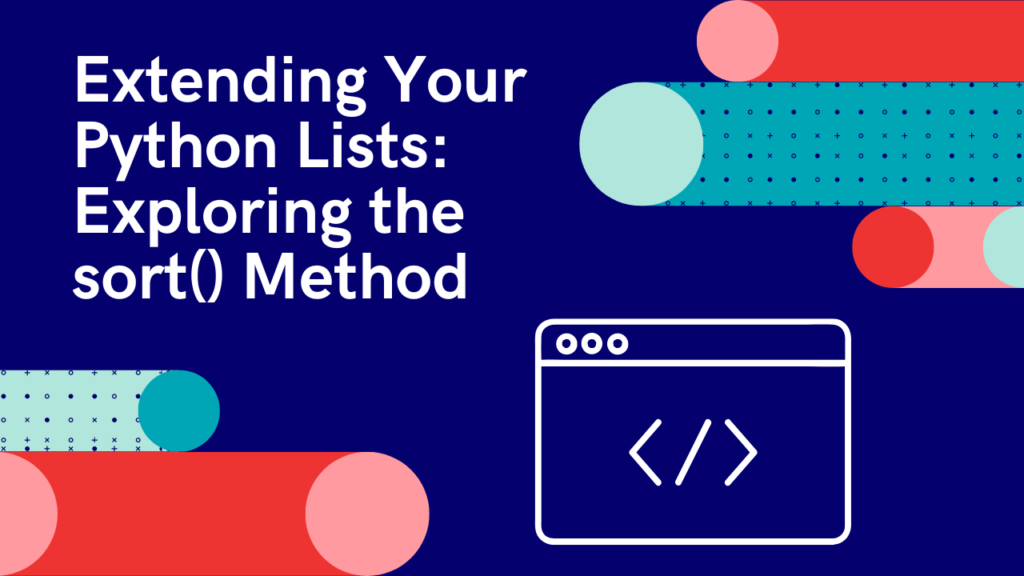
Introduction:
Python’s list data structure offers a variety of methods for efficient list manipulation. Among these methods, sort() stands out as a powerful tool for sorting the elements within a list in ascending or descending order. In this blog post, we will explore the sort() method in detail, uncovering its functionality, use cases, and providing practical examples to help you harness its power for effective list sorting.
Understanding sort():
The sort() method in Python allows us to sort the elements within a list in a specific order. By default, it sorts the list in ascending order, but it can also be used to sort in descending order by providing the reverse=True
argument.
Syntax:
The syntax for using the sort() method is as follows:
list_name.sort(reverse=False)
Here, list_name
represents the name of the list that we want to sort, and reverse
is an optional parameter. If reverse
is set to True
, the list is sorted in descending order. By default, reverse
is set to False
for ascending order sorting.
Sorting a List:
The sort() method provides a convenient way to arrange the elements within a list in a specific order. Let’s explore some examples to illustrate its usage:
Example 1: Sorting a List in Ascending Order
numbers = [5, 2, 8, 1, 7]
numbers.sort()
print(numbers)
Output:
[1, 2, 5, 7, 8]
In this example, we have a list of numbers, and by calling the sort() method, we arrange the elements in ascending order.
Example 2: Sorting a List in Descending Order
fruits = ["apple", "banana", "orange"]
fruits.sort(reverse=True)
print(fruits)
Output:
["orange", "banana", "apple"]
Here, we have a list of fruits, and by using the sort() method with the reverse=True
argument, we sort the elements in descending order.
Example 3: Sorting a List of Custom Objects
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
people = [Person("Alice", 25), Person("Bob", 20), Person("Charlie", 30)]
people.sort(key=lambda person: person.age)
for person in people:
print(person.name, person.age)
Output:
Bob 20
Alice 25
Charlie 30
In this example, we have a list of Person
objects, and we sort them based on the age
attribute using the key
parameter along with a lambda function.
Important Considerations:
- The sort() method modifies the list in place. It does not create a new sorted list but updates the existing list.
- The sort() method only works for homogeneous lists containing elements of the same type or comparable elements.
- When sorting custom objects, you can provide a
key
function that specifies the attribute or value to consider during the sorting process.
Tips for Effective Usage:
- Use the sort() method to arrange the elements within a list in a desired order, making it easier to search or analyze the data.
- If you want to preserve the original list while creating a sorted version, use the
sorted()
function instead of the sort() method.
Conclusion:
The sort() method in Python is a powerful tool for sorting the elements within a list. By understanding its syntax, usage, and important considerations, you now possess a valuable tool for effective list sorting. Utilize the sort() method effectively, and you’ll have greater control over arranging and organizing your lists in Python.
Happy coding!
The Education Machine
Leave a Reply