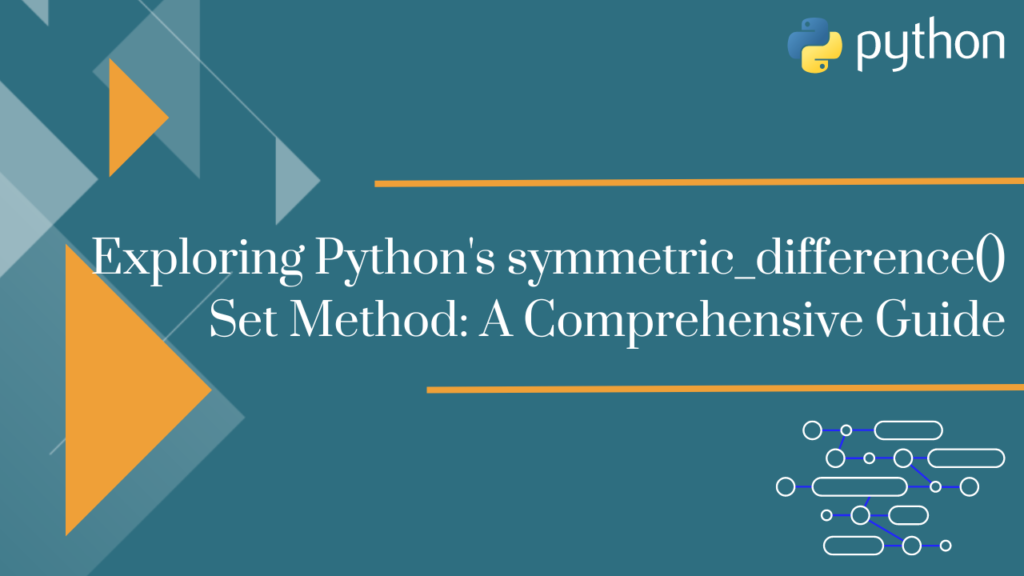
Introduction
In Python, the symmetric_difference() method is a powerful tool available for sets, allowing us to find the elements that exist in either of two sets, but not in both. By leveraging this method, we can efficiently perform set operations, identify unique elements, and analyze differences between sets. In this comprehensive guide, we will delve into the intricacies of the symmetric_difference() method, exploring its functionality, syntax, usage examples, and best practices.
Understanding the symmetric_difference() Method
The symmetric_difference() method is specifically designed for sets in Python. It returns a new set that contains elements present in either of the two sets, but not in both. In other words, it provides the symmetric difference between the sets.
Syntax of symmetric_difference()
The syntax for using the symmetric_difference() method is as follows:
set1.symmetric_difference(set2)
Here, set1 and set2 are the sets to be compared. The method returns a new set containing the symmetric difference between the two sets.
Exploring Examples
Let’s consider a few examples to better understand the usage and behavior of the symmetric_difference() method.
Example 1
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result = set1.symmetric_difference(set2)
print(result)
Output
{1, 2, 5, 6}
In this example, set1 and set2 have common elements 3 and 4. The symmetric_difference() method returns a new set containing the elements that are present in either set1 or set2, but not in both.
Example 2
set1 = {1, 2, 3}
set2 = {4, 5, 6}
result = set1.symmetric_difference(set2)
print(result)
Output
{1, 2, 3, 4, 5, 6}
Here, set1 and set2 have no common elements. Therefore, the symmetric_difference() method returns a new set containing all the elements from both sets.
Common Use Cases
The symmetric_difference() method finds application in various scenarios, such as:
- Identifying unique elements across multiple sets
- Analyzing differences between data sets or collections
- Performing exclusive operations based on set differences
- Filtering out common elements from sets to focus on unique values
Best Practices for Using symmetric_difference()
To ensure optimal usage of the symmetric_difference() method, consider the following best practices:
- Utilize sets when appropriate, as they provide faster membership tests and eliminate duplicates.
- Combine the symmetric_difference() method with other set methods like union() and intersection() for advanced set operations.
- Avoid modifying the original sets if preservation of the original data is required by assigning the result to a new set variable.
Conclusion
In this comprehensive guide, we have explored the symmetric_difference() method in Python sets, understanding its purpose, syntax, and examples. We have also examined common use cases and provided best practices for efficient utilization. By leveraging the power of symmetric_difference(), you can perform set operations, analyze differences between sets, and identify unique elements in your Python programs.
Remember to experiment with the symmetric_difference() method and incorporate it into your Python projects to take full advantage of its capabilities.
Happy coding!
The Education Machine
Leave a Reply